Building: TinyORM
- Introduction
- Folders structure
- Getting started
- vcpkg
- C preprocessor macros
- Building with CMake
- Building with qmake
- Ccache support
Introduction
The build systems supported out of the box are CMake
and qmake
.
All examples below assume that pwsh
runs on Windows
and bash
runs on Linux
.
Common Prerequisites
Install the required dependencies before starting.
The QSqlDatabase
depends on QCoreApplication
from Qt v6.5.3
so you must create the QCoreApplication
instance before you will call anything from the TinyORM
library. 🫤 The change was made here.
Windows Prerequisites
Build environment scripts
The Visual Studio
does not provide vcvars
scripts for pwsh
, you can use vcvars64.ps1
provided by TinyORM
in the tools/
folder. Place them on the $env:Path
user/system path and they will be available system-wide.
The same is true for the Qt Framework
, it doesn't provide qtenv
scripts for pwsh
too. You can create your own script, place it on the $env:Path
user/system path and it will be available system-wide.
Here is one simple example for pwsh
and bash
on Linux
.
- pwsh
- bash
#!/usr/bin/env pwsh
Set-StrictMode -Version 3.0
Write-Host 'Setting up environment for Qt 6.7.2 usage...' -ForegroundColor Magenta
Write-Host
$Script:QtRoot = $env:TINY_QT_ROOT ?? 'C:\Qt'
$env:Path = "$Script:QtRoot\6.7.2\msvc2019_64\bin;" + $env:Path
. vcvars64.ps1
#!/usr/bin/env sh
echo 'Setting up environment for Qt 6.7.2 usage...'
QtRoot="${TINY_QT_ROOT:-/opt/Qt}"
export PATH="$QtRoot/6.7.2/gcc_64/bin"${PATH:+:}$PATH
export LD_LIBRARY_PATH="$QtRoot/6.7.2/gcc_64/lib"${LD_LIBRARY_PATH:+:}$LD_LIBRARY_PATH
These scripts consider the TINY_QT_ROOT
environment variable that should point to the Qt
installation folder, you can define this environment variable globally in your OS.
You can't execute these qtenvX
scripts, they have to be sourced like source qtenvX
or . qtenvX
.
Allow symbolic links unprivileged
Open Local Security Policy
, go to Local Policies - User Rights Assignment
, open Create symbolic links
and add your user account or user group, restart when it doesn't apply immediately.
Folders structure
All tinyorm.org
examples are based on the following folders structure. The tom
folder will contain a migrations console application.
You can set the root and application folder paths in the form below and they will be used across the whole www.tinyorm.org website. 🥳 The pwsh shell is supposed to use on Windows and the bash shell on Linux, but it is not a requirement.
- pwsh
- bash
Current pwsh path
├──
│ ├── HelloWorld/
│ | ├── HelloWorld/
│ | ├── HelloWorld-builds-cmake/
│ | | └── build-debug/
│ | └── HelloWorld-builds-qmake/
│ | └── build-debug/
│ ├── TinyORM/
│ | ├── TinyORM/
│ | ├── TinyORM-builds-cmake/
│ | │ ├── build-gcc-debug/
│ | │ ├── build-gcc-release/
│ | │ └── build-clang-debug/
│ | └── TinyORM-builds-qmake/
│ | ├── build-debug/
│ | ├── build-TinyORM-Desktop_Qt_6_7_2_MSVC2019_64bit-Debug/
│ | └── build-TinyORM-Desktop_Qt_6_7_2_MSYS2_UCRT64_64bit-Release/
│ └── tom/
│ ├── tom/
│ │ └── database/
│ │ ├── migrations/
│ │ ├── seeders/
│ │ ├── migrations.pri
│ │ └── seeders.pri
│ ├── tom-builds-cmake/
│ │ └── build-TinyORM-Desktop_Qt_6_7_2_MSVC2019_64bit-Debug/
│ └── tom-builds-qmake/
│ ├── build-TinyORM-Desktop_Qt_6_7_2_MSYS2_UCRT64_64bit-Release/
│ └── build-TinyORM-Desktop_Qt_6_7_2_MSVC2019_64bit-Debug/
├── tmp/
└── vcpkg/
Current bash path
├──
│ ├── HelloWorld/
│ | ├── HelloWorld/
│ | ├── HelloWorld-builds-cmake/
│ | | └── build-debug/
│ | └── HelloWorld-builds-qmake/
│ | └── build-debug/
│ ├── TinyORM/
│ | ├── TinyORM/
│ | ├── TinyORM-builds-cmake/
│ | │ ├── build-gcc-debug/
│ | │ ├── build-gcc-release/
│ | │ └── build-clang-debug/
│ | └── TinyORM-builds-qmake/
│ | ├── build-debug/
│ | ├── build-TinyORM-Desktop_Qt_6_7_2_GCC_64bit-Debug/
│ | └── build-TinyORM-Desktop_Qt_6_7_2_clang18_64bit_ccache-Release/
│ └── tom/
│ ├── tom/
│ │ └── database/
│ │ ├── migrations/
│ │ ├── seeders/
│ │ ├── migrations.pri
│ │ └── seeders.pri
│ ├── tom-builds-cmake/
│ │ └── build-TinyORM-Desktop_Qt_6_7_2_clang18_64bit_ccache-Debug/
│ └── tom-builds-qmake/
│ ├── build-TinyORM-Desktop_Qt_6_7_2_GCC_64bit-Debug/
│ └── build-TinyORM-Desktop_Qt_6_7_2_clang18_64bit_ccache-Release/
├── tmp/
└── vcpkg/
Avoid paths with spaces with the qmake
build system, it will not compile.
You can force the QtCreator
to generate a build folders structure as is described above.
To generate the required folders structure set the Settings
- Build & Run
- Default Build Properties
- Default build directory
to:
../%{Project:Name}-builds-%{BuildSystem:Name}/%{JS: Util.asciify("build-%{Project:Name}-%{Kit:FileSystemName}-%{BuildConfig:Name}")}
Getting started
Prepare compilation environment, we need to put the Qt Framework and Visual Studio MSVC compiler on the path on Windows. The compiler is already on the path on Linux and you can export PATH
and LD_LIBRARY_PATH
for Qt Framework, or use our qtenvX
scripts described above.
- pwsh
- bash
mkdir
cd
$env:Path = 'C:\Qt\6.7.2\msvc2019_64\bin;' + $env:Path
vcvars64.ps1
mkdir -p
cd
export PATH=/opt/Qt/6.7.2/gcc_64/bin${PATH:+:}$PATH
export LD_LIBRARY_PATH=/opt/Qt/6.7.2/gcc_64/lib${LD_LIBRARY_PATH:+:}$LD_LIBRARY_PATH
You can also use the tools/Add-FolderOnPath.ps1
pwsh script to fastly prepend a path or pwd on the system PATH
.
vcpkg
Installing the vcpkg
is highly recommended, it simplifies installation of the range-v3
and tabulate
dependencies.
- pwsh
- bash
git clone git@github.com:microsoft/vcpkg.git
cd vcpkg
.\bootstrap-vcpkg.bat
git clone git@github.com:microsoft/vcpkg.git
cd vcpkg
./bootstrap-vcpkg.sh
Add vcpkg
on the system path, add the following to the .bashrc
or .zshrc
on Linux.
export PATH=/vcpkg${PATH:+:}$PATH
On Windows, open the Environment variables
dialog and add vcpkg
on the user PATH
.
Or you can export it for the current session only.
- pwsh
- bash
$env:Path = "\vcpkg;" + $env:Path
export PATH=/vcpkg${PATH:+:}$PATH
Set up vcpkg
environment
To export vcpkg
environment variables globally, add it to the .bashrc
or .zshrc
on Linux, and you can use the Environment variables
dialog on Windows.
export VCPKG_DEFAULT_TRIPLET=x64-linux
#export VCPKG_DEFAULT_HOST_TRIPLET=x64-linux
export VCPKG_MAX_CONCURRENCY=11
export VCPKG_OVERLAY_PORTS="$HOME/.local/share/vcpkg/ports"
export VCPKG_OVERLAY_TRIPLETS="$HOME/.local/share/vcpkg/triplets"
export VCPKG_ROOT="$HOME/Code/c/vcpkg"
It is recommended to define these variables globally because the CMake
and qmake
build system are able to detect the vcpkg
installation from them so you don't have to configure them manually to detect the vcpkg
installation.
On Windows, it's always better to create these types of variables as user variables instead of system variables in the Environment variables
dialog.
C preprocessor macros
The following table summarizes all the C preprocessor macros defined in the TinyORM
library. These C macros are configured by CMake
or qmake
build systems. They are not sorted alphabetically, but they are sorted by how significant they are.
In the CMake
build system, all the C macros are auto-detected / auto-configured or controlled by CMake build options
, so you don't have to care too much about them.
In the qmake
build is important whether you are building TinyORM
library or you are building your application and linking against TinyORM
library. When you are building the TinyORM
library, all the C macros are auto-detected / auto-configured or controlled by qmake build options
, so you don't have to care too much about them.
But a special situation is when you are building your application / library and you are linking against TinyORM
library. In this particular case, you must configure all these C macros manually! For this reason, the TinyOrm.pri
has been created, so that's not a big deal either. Little more info here.
C Macro Name | Description |
---|---|
TINYORM_LINKING_SHARED | Must be defined when you are linking against TinyORM shared build (dll library), exported classes and functions will be tagged with __declspec(dllimport) on msvc and visibility("default") on GCC >= 4 . |
TINYORM_BUILDING_SHARED | Defined when TinyORM is built as a dll library (shared build). |
TINYORM_DEBUG | Defined in the debug build. |
TINYORM_NO_DEBUG | Defined in the release build. |
TINYORM_DEBUG_SQL | Defined in the debug build. |
TINYORM_NO_DEBUG_SQL | Defined in the release build. |
TINYORM_MYSQL_PING | Enable Orm::MySqlConnection::pingDatabase() method.Defined when mysql_ping (qmake) / MYSQL_PING (cmake) configuration build option is enabled. |
TINYORM_DISABLE_ORM | Controls the compilation of all ORM-related source code, when this macro is defined , then only the query builder without ORM is compiled. Also excludes ORM-related unit tests.Defined when disable_orm (qmake) / ORM (cmake) configuration build option is enabled (qmake) / disabled (cmake). |
TINYORM_EXTERN_CONSTANTS | Defined when extern constants are used. Extern constants are enabled by default for shared builds and disabled for static builds. Described at qmake / CMake how it works. |
TINYORM_INLINE_CONSTANTS | Defined when global inline constants are used. Defined when inline_constants (qmake) / INLINE_CONSTANTS (cmake) configuration build option is enabled. |
TINYORM_TESTS_CODE | Enable code needed by unit tests, eg. connection overriding in the Orm::Tiny::Model .Defined when build_tests (qmake) / BUILD_TESTS (cmake) configuration build option is enabled. |
TINYORM_DISABLE_THREAD_LOCAL | Remove all thread_local storage duration specifiers, it disables multi-threading support.Defined when disable_thread_local (qmake) / DISABLE_THREAD_LOCAL (cmake) configuration build option is enabled. |
TINYTOM_MIGRATIONS_DIR | Default migrations path for the make:migration command, can be an absolute or relative path (to the pwd).Default value: database/migrations (relative to the pwd)Defined by TOM_MIGRATIONS_DIR (cmake) configuration build option.(qmake note) You can use DEFINES += TINYTOM_MIGRATIONS_DIR="\"database/migrations\"" on the command-line or set it in the main conf.pri file. |
TINYTOM_MODELS_DIR | Default models path for the make:model command, can be an absolute or relative path (to the pwd).Default value: database/models (relative to the pwd)Defined by TOM_MODELS_DIR (cmake) configuration build option.(qmake note) You can use DEFINES += TINYTOM_MODELS_DIR="\"database/models\"" on the command-line or set it in the main conf.pri file. |
TINYTOM_SEEDERS_DIR | Default seeders path for the make:seeder command, can be an absolute or relative path (to the pwd).Default value: database/seeders (relative to the pwd)Defined by TOM_SEEDERS_DIR (cmake) configuration build option.(qmake note) You can use DEFINES += TINYTOM_SEEDERS_DIR="\"database/seeders\"" on the command-line or set it in the main conf.pri file. |
TINYORM_USING_PCH | Defined if building with precompiled headers. Controlled by qmake / CMake . |
Building with CMake
If something is not clear, you can still look at GitHub Action workflows
how a building is done.
First, create a basic folder structure and then clone the TinyORM
project.
- pwsh
- bash
cd
mkdir /TinyORM/TinyORM-builds-cmake/build-debug
cd /TinyORM
git clone git@github.com:silverqx/TinyORM.git
cd
mkdir -p /TinyORM/TinyORM-builds-cmake/build-debug
cd /TinyORM
git clone git@github.com:silverqx/TinyORM.git
Configure & Build (cmake)
Now you are ready to configure the TinyORM
library.
cd TinyORM-builds-cmake/build-debug
- pwsh
- bash
cmake.exe `
-S "/TinyORM/TinyORM" `
-B "/TinyORM/TinyORM-builds-cmake/build-debug" `
-G 'Ninja' `
-D CMAKE_BUILD_TYPE:STRING='Debug' `
-D CMAKE_TOOLCHAIN_FILE:FILEPATH="/vcpkg/scripts/buildsystems/vcpkg.cmake" `
-D CMAKE_CXX_SCAN_FOR_MODULES:BOOL=OFF `
-D CMAKE_INSTALL_PREFIX:PATH="/tmp/TinyORM" `
-D BUILD_TESTS:BOOL=OFF `
-D MATCH_EQUAL_EXPORTED_BUILDTREE:BOOL=ON `
-D MYSQL_PING:BOOL=OFF `
-D TOM:BOOL=ON `
-D TOM_EXAMPLE:BOOL=OFF `
-D VERBOSE_CONFIGURE:BOOL=ON
cmake \
-S "/TinyORM/TinyORM" \
-B "/TinyORM/TinyORM-builds-cmake/build-debug" \
-G 'Ninja' \
-D CMAKE_BUILD_TYPE:STRING='Debug' \
-D CMAKE_TOOLCHAIN_FILE:FILEPATH="/vcpkg/scripts/buildsystems/vcpkg.cmake" \
-D CMAKE_CXX_SCAN_FOR_MODULES:BOOL=OFF \
-D CMAKE_INSTALL_PREFIX:PATH="/tmp/TinyORM" \
-D VERBOSE_CONFIGURE:BOOL=ON \
-D BUILD_TESTS:BOOL=OFF \
-D MYSQL_PING:BOOL=OFF \
-D TOM:BOOL=ON \
-D TOM_EXAMPLE:BOOL=OFF \
-D MATCH_EQUAL_EXPORTED_BUILDTREE:BOOL=ON
CMake STRICT_MODE
option
The STRICT_MODE
CMake
configuration option was added in TinyORM
v0.37.3
. This option was added to avoid the propagation of aggressive strict warning compiler/linker options and Qt definitions from the TinyORM
library to user code through the TinyOrm::CommonConfig
interface library.
TinyORM
uses the strictest warning level options, virtually anything that can be enabled is enabled to produce a better code. I highly recommend enabling this option to produce better code and to follow good practices. It also helps to follow the ISOCPP
C++ Core Guidelines standards.
If you want to enable these strict warning options in your code, you can enable the STRICT_MODE
CMake
configuration option and they will be propagated to your code. You can also enabled it globally using the TINYORM_STRICT_MODE
environment variable, and the value of this environment variable will be picked up during initial CMake configuration as the default value for the STRICT_MODE
CMake
configuration option.
You can achieve the same result by manually linking against the TinyOrm::CommonConfig
interface library when the STRICT_MODE
is set to OFF
.
target_link_libraries(<target> PRIVATE TinyOrm::CommonConfig)
The recommended way is to set the TINYORM_STRICT_MODE
environment variable to 1
or ON
.
Build TinyORM
And build. You don't have to install it, you can use the build tree directly if you want.
cmake --build . --target all
cmake --install .
Or build and install in one step.
cmake --build . --target install
CMake multi-config generators like Ninja Multi-Config
or Visual Studio 16 2019
are also supported.
CMake build options
Advanced TinyORM
options.
Important CMake
options.
Consume TinyOrm library (cmake)
In your application or library CMakeLists.txt
file add following find_package()
call.
find_package(TinyOrm 0.37.3 CONFIG REQUIRED)
If the TinyORM
build tree is not exported to the CMake's User Package Registry
then also add the TinyORM
build tree or CMAKE_INSTALL_PREFIX
folder to the CMAKE_PREFIX_PATH
, so CMake can find TinyORM's package configuration file during find_package(TinyOrm)
call.
- cmake (pwsh)
- cmake (bash)
# build tree
list(APPEND CMAKE_PREFIX_PATH "/TinyORM/TinyORM-builds-cmake/build-debug")
# installation folder - CMAKE_INSTALL_PREFIX
list(APPEND CMAKE_PREFIX_PATH "/tmp/TinyORM")
# build tree
list(APPEND CMAKE_PREFIX_PATH "/TinyORM/TinyORM-builds-cmake/build-debug")
# installation folder - CMAKE_INSTALL_PREFIX
list(APPEND CMAKE_PREFIX_PATH "/tmp/TinyORM")
Or as an alternative, you can set CMAKE_PREFIX_PATH
environment variable.
As the last thing, do not forget to add TinyOrm0d.dll
on the path on Windows and on the LD_LIBRARY_PATH
on Linux, so your application can find it during execution.
- pwsh
- bash
$env:Path = "\TinyORM\TinyORM-builds-cmake\build-debug;" + $env:Path
export LD_LIBRARY_PATH=/TinyORM/TinyORM-builds-cmake/build-debug${PATH:+:}$PATH
Now you can try the HelloWorld CMake
example.
You can also try the FetchContent
method to fastly link against the TinyORM
library.
Building with qmake
First, create a basic folder structure and then clone the TinyORM
project.
- pwsh
- bash
cd
mkdir /TinyORM/TinyORM-builds-qmake
cd /TinyORM
git clone git@github.com:silverqx/TinyORM.git
cd
mkdir -p /TinyORM/TinyORM-builds-qmake
cd /TinyORM
git clone git@github.com:silverqx/TinyORM.git
Install dependencies
With the qmake
build system, you have to install TinyORM
dependencies manually. We will use the vcpkg
package manager.
cd ../../vcpkg
vcpkg search range-v3
vcpkg search tabulate
vcpkg install range-v3 tabulate
vcpkg list
On Linux
, you can install the range-v3
library and some other dependencies with the package manager.
Configure & Build (qmake)
Open QtCreator IDE
I recommend creating a new Session
in the QtCreator
, this way you will have all the examples in one place and as a bonus, everything will be in the same place when you close and reopen QtCreator IDE
. You can name it tinyorm.org
or TinyORM examples
, it is up to you.
If you are using sessions, you can use a single clangd
instance for all projects in this session in the QtCreator IDE
. One significant advantage of this method is that the .qtc_clangd/
folder will not be created in the build folder, but will be stored globally in the Roaming profile. You can enable it in the Settings
- C++
- Clangd
- Sessions with a single clangd instance
.
Configure TinyORM
Now you are ready to configure the TinyORM
library. There are two ways how to configure the TinyORM
library and it's the new Auto-configure
feature added in TinyORM
v0.34.0
using the .env
files and the old way using the conf.pri
files.
Auto-configuration and tiny_dotenv
This is the new recommended method to auto-configure TinyORM's qmake
build system and also the dependencies, it was added in TinyORM
v0.34.0
. You need to copy the prepared .env.(win32|unix|mingw).example
file to the .env.(win32|unix|mingw)
. One .env
example file is prepared for each supported platform.
All prepared .env.(win32|unix|mingw).example
files are simple and clear. You can also create a common .env
file that is included before the platform-specific .env.(win32|unix|mingw)
files.
- pwsh
- bash
cd /TinyORM/TinyORM
cp .env.win32.example .env.win32
cd /TinyORM/TinyORM
cp .env.unix.example .env.unix
And that is all, if you have correctly set all qmake
variables in this .env.(win32|unix|mingw)
file or you have correctly set environment variables, then the qmake
build system should be able to auto-detect
all dependencies . 🔥
The Auto-configuration
and Environment files
internals are described at the end to make this section more clear.
The Auto-configuration
feature can be turned off using the disable_autoconf
qmake
configuration option (eg. CONFIG*=disable_autoconf
).
The tiny_dotenv
feature can be turned off using the disable_dotenv
qmake
configuration option (eg. CONFIG*=disable_dotenv
).
Manual configuration (conf.pri)
This is the old method used before TinyORM
v0.34.0
. You need to copy the conf.pri.example
files to conf.pri
(there are four, one for every project or sub-project) and manually update the INCLUDEPATH
and LIBS
to configure TinyORM's qmake
build dependencies. This way you can override any qmake
build options or variables.
To disable the Auto-configuration
feature you must define the disable_autoconf
qmake
configuration option (eg. CONFIG*=disable_autoconf
) because from TinyORM
v0.34.0
is the Auto-configuration
feature enabled by default.
You can also remove all .env
files or turn off the tiny_dotenv
feature using CONFIG*=disable_dotenv
. You can use them all at once if you want, .env
and also conf.pri
files.
conf.pri
files are nicely commented on, so you can see what needs to be modified.
- pwsh
- bash
cd /TinyORM/TinyORM
cp conf.pri.example conf.pri
cp tests/conf.pri.example tests/conf.pri
cp tests/testdata_tom/conf.pri.example tests/testdata_tom/conf.pri
cp examples/tom/conf.pri.example examples/tom/conf.pri
cd /TinyORM/TinyORM
cp conf.pri.example conf.pri
cp tests/conf.pri.example tests/conf.pri
cp tests/testdata_tom/conf.pri.example tests/testdata_tom/conf.pri
cp examples/tom/conf.pri.example examples/tom/conf.pri
The Manual configuration
internals are described at the end to make this section more clear.
The Manual configuration
is still relevant if you have any non-standard installation of the vcpkg
or MySQL
and the Auto-configuration
feature fails.
Opening TinyORM.pro (main project file)
Now you can open the TinyORM.pro
project in the QtCreator IDE
.
This will open the Configure Project
tab, select some kit and update build folder paths to meet our folders structure or like you want.
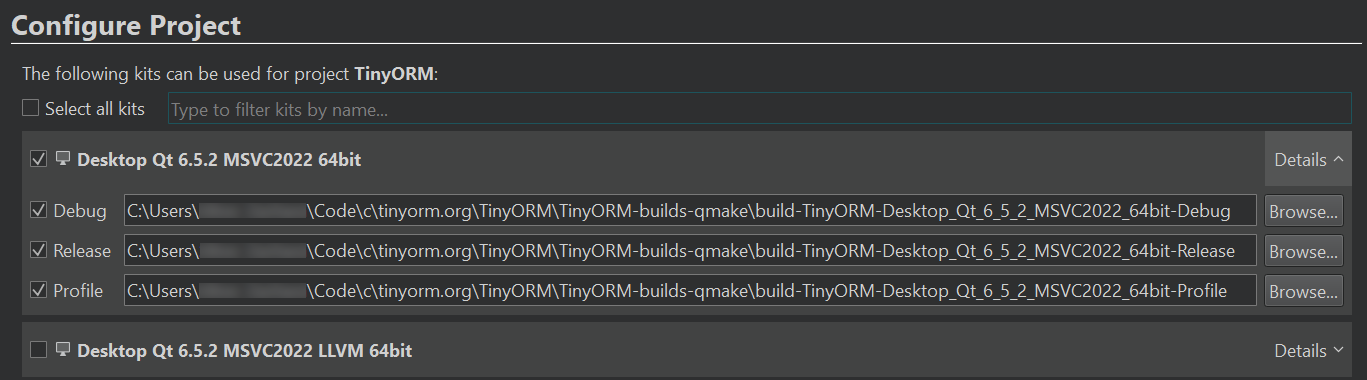
You can force the QtCreator
to generate a build folders structure as is described above.
You are ready to configure build options, hit Ctrl+5 to open Project Settings
tab and select Build
in the left sidebar to open the Build Settings
, it should look similar to the following picture.
Disable QML debugging and profiling
and Qt Quick Compiler
, they are not used.
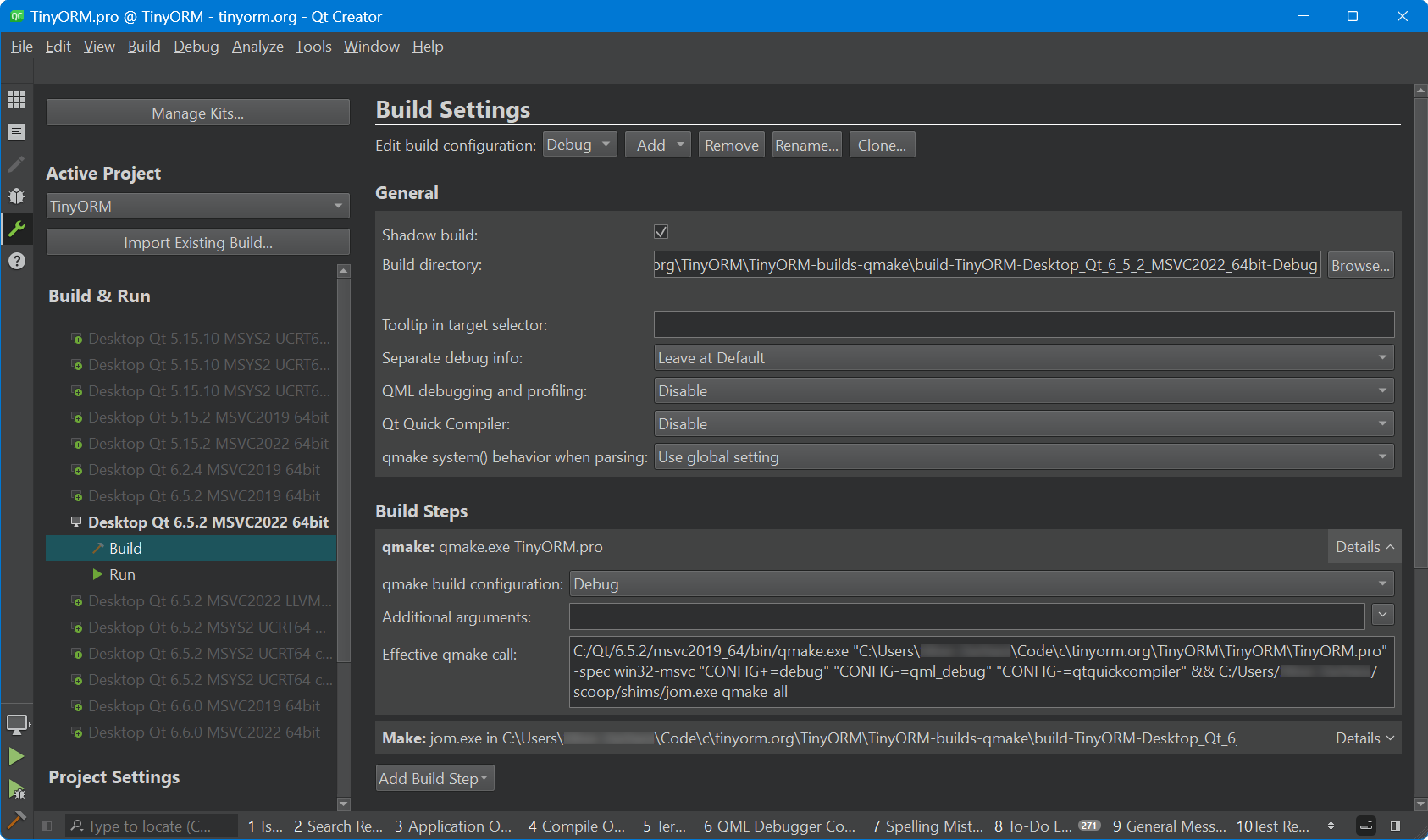
If you want to change some TinyORM
build options, you can pass them to the Build Steps
- qmake TinyORM.pro
- Additional arguments
input field. It can look like this.

Build TinyORM
Everything is ready for build, you can press Ctrl+b to build the project.
qmake build options
Advanced TinyORM
options.
Important qmake
options.
Consume TinyOrm library (qmake)
The TinyOrm.pri
file is available to simplify the integration of the TinyORM
library into your application. It sets up and configures the CONFIG
and DEFINES
qmake variables, adds the TinyORM
, tom
, and vcpkg
header files on the system INCLUDEPATH
(cross-platform using the -isystem
or -imsvc
), links against the TinyORM shared
or static
library using the LIBS
.
You can use it to configure the TinyORM
library when you are linking against it. It does a very similar thing like the CMake's Find Modules
feature.
Requirements
It has a few requirements, you need to:
- specify path to the
TinyORM
qmake features (.prf
files) using theQMAKEFEATURES
variable that can only be set in the.qmake.conf
file - specify
qmake
orenvironment
variables to find thevcpkg
installation (TINY_VCPKG_ROOT
andTINY_VCPKG_TRIPLET
) - specify path to the
TinyORM
build folder (TINYORM_BUILD_TREE
)- you can specify it manually
- or you can use Partial guessing of the
TINYORM_BUILD_TREE
- build your application with the same
CONFIG
qmake
variables that were used when building theTinyORM
library
Let's explain one by one.
QMAKEFEATURES
Create the .qmake.conf
file in your application root folder with the following content.
# Path to the PARENT folder of the TinyORM source folder
TINY_MAIN_DIR = $$clean_path(<your_path>)
# To find .env and .env.$$QMAKE_PLATFORM files in YOUR project
TINY_DOTENV_ROOT = $$PWD
# Path to the TinyORM build folder (specified manually)
TINYORM_BUILD_TREE = $$quote($$TINY_MAIN_DIR/TinyORM-builds-qmake/build-TinyORM-Desktop_Qt_6_7_2_MSVC2019_64bit-Debug/)
# vcpkg - range-v3 and tabulate
TINY_VCPKG_ROOT = $$quote(<your_path>/vcpkg/)
#TINY_VCPKG_TRIPLET = x64-windows
# To find .prf files, needed by eg. CONFIG += tiny_system_headers inline/extern_constants
QMAKEFEATURES *= $$quote($$TINY_MAIN_DIR/TinyORM/qmake/features)
You can move all qmake
variables that are part of the qmake
configuration process to the .env
file if you want (recommended), this is possible because the TinyOrm.pri
enables the Environment files
feature by default.
You can look at the Auto-configure using .qmake.conf and .env example for Hello world
project of what must stay in the qmake.conf
file and what can be moved to the .env
files.
You can use the Partial guessing of the TINYORM_BUILD_TREE
if you don't like to specify it manually.
Variables affecting TinyOrm.pri
You must define the following variables before the TinyOrm.pri
is included:
Variable Name | Description |
---|---|
TINYORM_BUILD_TREE | Path to the TinyORM build folder. |
TINY_VCPKG_ROOT | Path to the vcpkg installation folder.If not defined, then it tries to use the VCPKG_ROOT environment variable. |
TINY_VCPKG_TRIPLET | The vcpkg triplet to use (vcpkg/installed/$$TINY_VCPKG_TRIPLET/).If not defined, then it tries to guess the vcpkg triplet based on the current compiler and OS (based on the QMAKESPEC ), and as the last thing, it tries to use the VCPKG_DEFAULT_TRIPLET environment variable. |
These variables will be set after the configuration is done:
Variable Name | Description |
---|---|
TINY_BUILD_SUBFOLDER | Folder by release type if CONFIG+=debug_and_release is defined (/debug, /release, or an empty string). |
TINY_CCACHE_BUILD | To correctly link ccache build against a ccache build (_ccache or an empty string). |
TINY_MSVC_VERSION | The MSVC compiler string (MSVC2022 or MSVC2019). |
TINY_QT_VERSION_UNDERSCORED | Underscored Qt version (eg. 6_7_2). |
TINY_RELEASE_TYPE_CAMEL | Build type string (Debug, Profile, or Release). |
TINY_VCPKG_INCLUDE | Path to the vcpkg include folder (vcpkg/installed/<triplet>/include/). |
Then you simply include the TinyOrm.pri
in your project file.
include($$TINY_MAIN_DIR/TinyORM/qmake/TinyOrm.pri)
And that is all, now you should be able to link against the TinyORM
library. 👌
Manual configuration examples
Frankly, there is no reason to use the Manual configuration (define the variables described below before the TinyOrm.pri
inclusion), the only reason to use it is when you want more control over this process or want to define everything yourself. I'll leave this section here to show how things work.
You will have to link against the TinyORM
library manually if you don't set the TINYORM_BUILD_TREE
qmake
variable before the inclusion of the TinyOrm.pri
file. The INCLUDEPATH
is auto-detected every time.
- pwsh
- bash
# Link against TinyORM library
# ---
TINY_MAIN_DIR = $$clean_path(<your_path>)
# Configure TinyORM library
include($$TINY_MAIN_DIR/TinyORM/qmake/TinyOrm.pri)
# TinyORM library path
TINYORM_BUILD_TREE = $$quote($$TINY_MAIN_DIR/TinyORM-builds-qmake)
LIBS += $$quote(-L$$TINYORM_BUILD_TREE/build-TinyORM-Desktop_Qt_6_7_2_MSVC2019_64bit-Debug/src$${TINY_BUILD_SUBFOLDER}/)
LIBS += -lTinyOrm
# Link against TinyORM library
# ---
TINY_MAIN_DIR = $$clean_path(<your_path>)
# Configure TinyORM library
include($$TINY_MAIN_DIR/TinyORM/qmake/TinyOrm.pri)
# TinyORM library path
TINYORM_BUILD_TREE = $$quote($$TINY_MAIN_DIR/TinyORM-builds-qmake)
LIBS += $$quote(-L$$TINYORM_BUILD_TREE/build-TinyORM-Desktop_Qt_6_7_2_GCC_64bit-Debug/src$${TINY_BUILD_SUBFOLDER}/)
LIBS += -lTinyOrm
The same is true for the vcpkg
include path. If you don't set the TINY_VCPKG_ROOT
or have not defined the VCPKG_ROOT
environment variable, then you need to set up the INCLUDEPATH
for the vcpkg
that provides the range-v3
and tabulate
header files.
- pwsh
- bash
# vcpkg - range-v3 and tabulate
# ---
INCLUDEPATH += $$quote(<your_path>/vcpkg/installed/x64-windows/include/)
# vcpkg - range-v3 and tabulate
# ---
QMAKE_CXXFLAGS += -isystem $$shell_quote(<your_path>/vcpkg/installed/x64-linux/include/)
You can also use TinyORM's qmake
function tiny_add_system_includepath()
which handles INCLUDEPATH
in a cross-platform way.
# vcpkg - range-v3 and tabulate
# ---
load(private/tiny_system_includepath)
tiny_add_system_includepath(<your_path>/vcpkg/installed/x64-linux/include/)
Do not forget to add TinyOrm0.dll
on the path on Windows and on the LD_LIBRARY_PATH
on Linux, so your application can find it during execution.
- pwsh
- bash
$env:Path = "\TinyORM\TinyORM-builds-qmake\build-debug;" + $env:Path
export LD_LIBRARY_PATH=/TinyORM/TinyORM-builds-qmake/build-debug${PATH:+:}$PATH
On Linux -isystem
marks the directory as a system directory, it prevents warnings.
On Windows you can use QMAKE_CXXFLAGS_WARN_ON = -external:anglebrackets -external:W0
, it applies a warning level 0 to the angel bracket includes; #include <file>
.
With the Clang-cl
with MSVC
you can use -imsvc
.
Auto-configuration internals
The qmake
build system does not support auto-configuration
of dependencies out of the box but TinyORM
from v0.34.0
added its own Auto-configuration
feature along with the tiny_dotenv
qmake feature. These new features allow us to auto-configure
TinyORM
project, and with their help, the conf.pri
files can be skipped entirely.
While it adds additional complexity to the qmake
configuration process, the benefits are significant.
The Auto-configuration
feature is designed to find the vcpkg
and MySQL
installations, and tiny_dotenv
to include the .env
and .env.(win32|unix|mingw)
files in the project's root folder. These new features can be configured using qmake
and environment
variables, and they also contain some guessing logic if these variables are not defined.
The Auto-configuration
feature can be turned off using the disable_autoconf
qmake
configuration option (eg. CONFIG*=disable_autoconf
).
These are qmake
and environment
variables that affect the Auto-configuration
feature:
Variable Name | Description |
---|---|
TINY_VCPKG_ROOT | Path to the vcpkg installation folder.If not defined, then it tries to use the VCPKG_ROOT environment variable. |
TINY_VCPKG_TRIPLET | The vcpkg triplet to use (vcpkg/installed/$$TINY_VCPKG_TRIPLET/).If not defined, then it tries to guess the vcpkg triplet based on the current compiler and OS (based on the QMAKESPEC ), and as the last thing, it tries to use the VCPKG_DEFAULT_TRIPLET environment variable. |
TINY_MYSQL_ROOT | Path to the MySQL installation folder.If not defined, then it tries to guess the MySQL installation folder (win32 only): $$(ProgramFiles)/MySQL/MySQL Server (8.4|8.3|8.2|8.1|8.0|5.7)/ |
You can set these variables in the .env
(recommended) or conf.pri
files, in the .qmake.conf
file (or wherever you want), or as environment variables.
These variables will be set after auto-configuration
is done:
Variable Name | Description |
---|---|
TINY_VCPKG_INCLUDE | Path to the vcpkg include folder (vcpkg/installed/<triplet>/include/). |
TINY_MYSQL_INCLUDE | Path to the MySQL include folder (MySQL Server 8.4/include/). |
TINY_MYSQL_LIB | Path to the MySQL lib folder (MySQL Server 8.4/lib/). |
The TINY_MYSQL_INCLUDE
and TINY_MYSQL_LIB
are only set on win32
platform except mingw
.
Environment files
The tiny_dotenv
feature allows us to define the .env
and .env.$$TINY_DOTENV_PLATFORM
files in the project's root folder. These files are loaded as early as possible so you can affect the qmake
configuration process. On the other hand, the conf.pri
files are loaded as late as possible, and they can be used to override the qmake
configuration.
The .env
file is included first and is included on all platforms.
There is only one requirement for this feature to work correctly, and that is to set the TINY_DOTENV_ROOT
qmake
variable to the project's root folder. This variable is already set in the .qmake.conf
file for the TinyORM
project.
Then the following names are taken into account: .env, .env.win32, .env.unix, .env.mingw
# To find .env and .env.$$QMAKE_PLATFORM files
TINY_DOTENV_ROOT = $$PWD
The tiny_dotenv
feature can be turned off using the disable_dotenv
qmake
configuration option (eg. CONFIG*=disable_dotenv
).
Environment files
don't work in the CMake
builds.
Partial guessing of the TINYORM_BUILD_TREE
You don't have to manually define the TINYORM_BUILD_TREE
in .env
or .qmake.conf
files. The TINYORM_BUILD_TREE
absolute path can be put together for you (this is happening inside the variables.pri
file) and TinyORM
build folder name can be guessed for you too.
You must define the following variables before the TinyOrm.pri
will be included to make this real (set them in the .qmake.conf
):
Variable Name | Description |
---|---|
TINY_MAIN_DIR | Path to the PARENT folder of the TinyORM source folder. |
TINY_BUILD_TREE | Path to the current build tree - TINY_BUILD_TREE = $$shadowed($$PWD) . |
The TINY_MAIN_DIR
is required for another features anyway (so it should already be set) and all that's left is to set the TINY_BUILD_TREE
.
# Path to the current build tree (used to guess the TinyORM build tree)
TINY_BUILD_TREE = $$shadowed($$PWD)
If you will follow this pattern or logic then you can switch QtCreator Kits
and the TINYORM_BUILD_TREE
will be auto-generated correctly and will always point to the correct TinyORM
build tree.
It works this way, all is happening inside the variables.pri
, it takes a build folder name for the current project eg. build-HelloWorld-Desktop_Qt_6_7_2_MSVC2022_64bit-Debug
, replaces the HelloWorld
with the TinyORM
and as we already know the TinyORM
build folder location we can simply concatenate these paths like $$TINY_MAIN_DIR/TinyORM-builds-qmake/build-TinyORM-Desktop_Qt_6_7_2_MSVC2022_64bit-Debug
.
This will only work if you follow the recommended Folders structure
.
Manual configuration internals
There is not much to say about the Manual configuration
feature. It uses conf.pri
files (there are four, one for every project or sub-project), and every project has prepared its own conf.pri.example
file for faster initial configuration.
These conf.pri.example
files are nicely commented on, so you can see what needs to be modified. The conf.pri
files are loaded as late as possible, and they can be used to override the qmake
configuration.
If the Auto-configuration
feature is disabled and there are no conf.pri
files, then the TinyORM
qmake
configuration or build will fail at 100%.
These conf.pri
files are intended for configuring qmake's INCLUDEPATH
and LIBS
, CONFIG
or eg. QMAKE_LFLAGS
, or any other qmake
options or variables.
Ccache support
The TinyORM supports the ccache
out of the box for all supported compilers. For qmake
you can enable it using the CONFIG+=ccache
and for CMake
you can set the CMAKE_CXX_COMPILER_LAUNCHER=ccache
.
On Linux
it's clear, the ccache
is fully supported and works also with the precompiled headers
. But was necessary to add some workarounds to the qmake
/CMake
build systems to make out of the box support on Windows
. When you enable the ccache
on Windows
then the build system replaces the -Zi
and -ZI
compiler options with the -Z7
(link to the issue) and disables precompiled headers
if ccache <4.10
.
You can install the ccache
using the scoop install ccache
command on Windows. See the Dependencies page for how to install ccache
on Linux.