Database: Migrations
- Introduction
- Generating Migrations
- Tab completion
- Migration Structure
- Running Migrations
- Tables
- Columns
- Indexes
Introduction
Migrations are like version control for your database, allowing your team to define and share the application's database schema definition. If you have ever had to tell a teammate to manually add a column to their local database schema after pulling in your changes from source control, you've faced the problem that database migrations solve.
The TinyORM Schema
facade provides database agnostic support for creating and manipulating tables across all of TinyORM's supported database systems. Typically, migrations will use this facade to create and modify database tables and columns.
The tom
migrations is a small console application that depends on the TinyORM
library. All migrations logic is compiled so recompilation is needed after adding a new migration class.
The schema builder
and migrations
don't support multi-threading.
TinyORM's schema builder supports all supported databases out of the box.
The following image shows what the tom
console application looks like. ✨ As you can see it offers everything that is needed to generate and manage migrations and seeders and all of this is backed up with the tab completion.
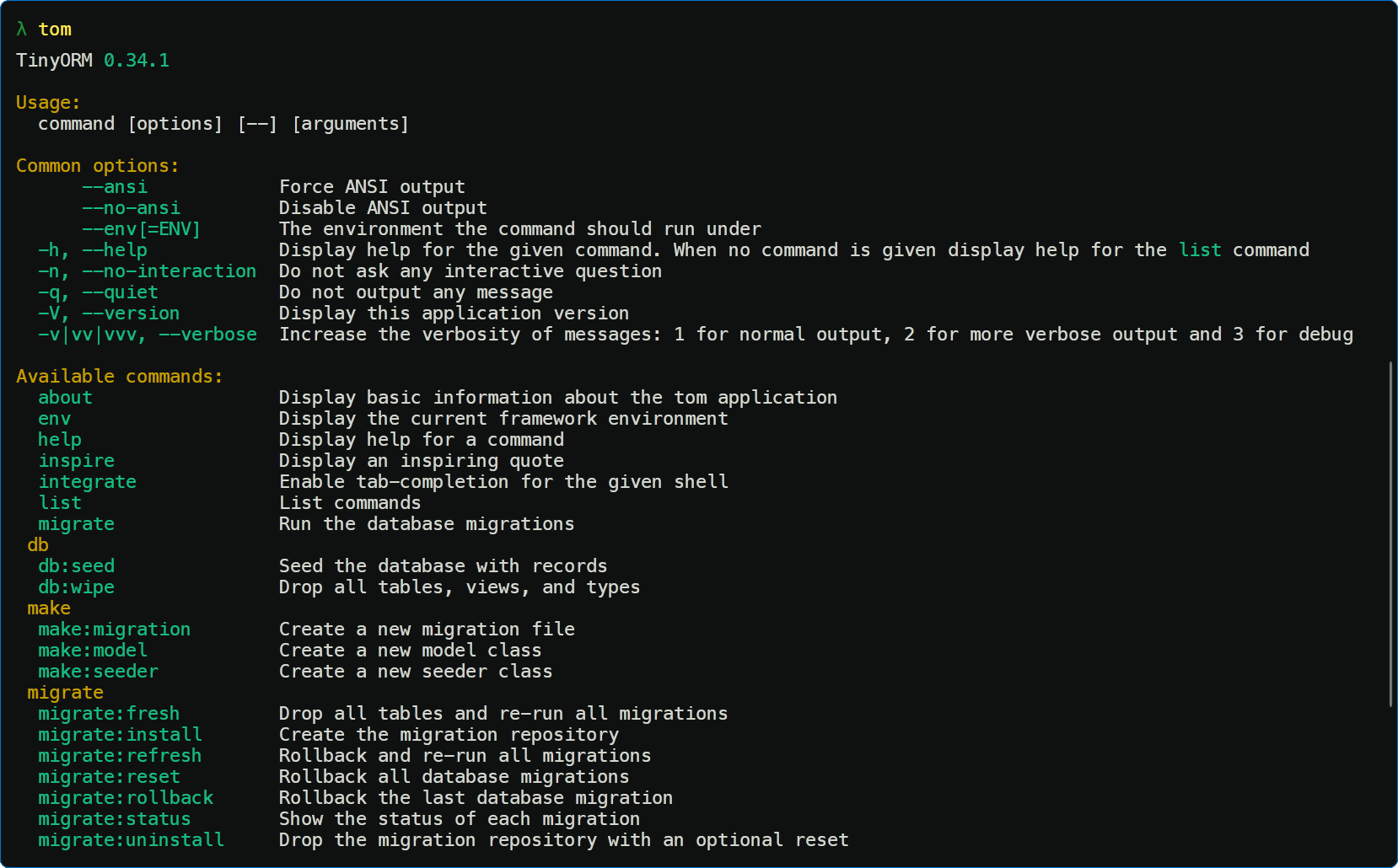
Generating Migrations
You may use the make:migration
tom
command to generate a database migration. The new migration will be placed in your database/migrations
directory. Each migration filename contains a timestamp that allows tom
to determine the order of the migrations:
tom make:migration create_posts_table
tom will use the name of the migration to attempt to guess the name of the table and whether or not the migration will be creating a new table. If tom
is able to determine the table name from the migration name, tom
will pre-fill the generated migration file with the specified table. Otherwise, you may simply specify the table in the migration file manually.
If you would like to specify a custom path for the generated migration, you may use the --path
option when executing the make:migration
command. The given path should be relative to your pwd or you can use the --realpath
option and pass the absolute path to the --path
option.
Migrations naming rules
If the migration name starts with the create_
string then the stub for table creation will be used and if the migration name contains _(from|to|in)_
then the stub for table update will be used. You can override these rules using the --create
and --table
options and specify the table name manually.
You can also pass the full migration filename with the datetime prefix and extension to the make:migration
. This command is able to detect almost any combination of the passed value, with or without datetime prefix or extension if it is the filename; or StudlyCase, snake_case, or kebab-case if it is the classname or any combination described above. 👀
Tab completion
Tab completion is available for the pwsh
(on Linux too), bash
, and zsh
shells. For pwsh
the tom.exe
and TinyOrm0.dll
library must be on the system path to work properly. With bash
if the tom
executable and libTinyOrm.so
library is not on the system path then it will provide less accurate completions.
You can enable it using the following commands.
- pwsh
- bash
- zsh
tom integrate pwsh
tom integrate bash
tom integrate zsh
tom integrate zsh --path=/usr/share/zsh/site-functions
Or you can enable it manually. Following actions are the same as the tom integrate
command does.
For the pwsh
paste the following code to the pwsh profile (works on Linux or Windows).
Register-ArgumentCompleter -Native -CommandName tom,tom_testdata -ScriptBlock {
Param($wordToComplete, $commandAst, $cursorPosition)
[Console]::InputEncoding =
[Console]::OutputEncoding = $OutputEncoding = [System.Text.Utf8Encoding]::new()
$Local:word = $wordToComplete.Replace('"', '\"')
$Local:ast = $commandAst.ToString().Replace('"', '\"')
tom complete --word="$Local:word" --commandline="$Local:ast" --position=$cursorPosition
| ForEach-Object {
$completionText, $listText, $toolTip = $_ -split ';', 3
$listText ??= $completionText
$toolTip ??= $completionText
[System.Management.Automation.CompletionResult]::new(
$completionText, $listText, 'ParameterValue', $toolTip)
}
}
For bash
you can copy or create symlink of the /tools/completions/tom.bash
file to the /usr/share/bash-completion/completions
folder.
sudo ln -s /TinyORM/tools/completions/tom.bash /usr/share/bash-completion/completions/tom
And for zsh
you can copy or create symlink of the /tools/completions/tom.zsh
file to the _tom
file to /usr/local/share/zsh/site-functions
folder.
sudo ln -s /TinyORM/tools/completions/tom.zsh /usr/local/share/zsh/site-functions/_tom
It will provide completions for the tom
commands, long and short parameters, and also for some positional arguments like namespaces for the list
command or commands for the help
command.
The tom integrate zsh
command also accepts the --path=
option with which you can set the location, where the zsh completion file should be created.
You can also output the completion script using the --stdout
option eg. tom integrate bash --stdout
.
Alternative installation methods
This section describes alternative installation methods for bash
and zsh
tab completions.
Static installation
Idea is to output the tab completion to the file and then source it.
- bash
- zsh
mkdir -p ~/.local/share/tom
tom integrate bash --stdout > ~/.local/share/tom/tom.bash
# Then source this file in the ~/.bashrc
source $HOME/.local/share/tom/tom.bash
mkdir -p ~/.local/share/tom
tom integrate zsh --stdout > ~/.local/share/tom/tom.zsh
# Then source this file in the ~/.zshrc
source $HOME/.local/share/tom/tom.zsh
compdef _tom tom
Dynamic installation
Idea is to avoid outputting the tab completion to the file, so you eval
the tab completion source code right away.
- bash
- zsh
# Add this eval to the ~/.bashrc
eval "$(tom integrate bash --stdout)"
# Add this eval to the ~/.zshrc
eval "$(tom integrate zsh --stdout)"
compdef _tom tom
Migration Structure
A migration class contains two methods: up
and down
. The up
method is used to add new tables, columns, or indexes to your database, while the down
method should reverse the operations performed by the up
method.
Within both of these methods, you may use the TinyORM schema builder to expressively create and modify tables. To learn about all of the methods available on the Schema
builder, check out its documentation. For example, the following migration creates a posts
table:
#pragma once
#include <tom/migration.hpp>
namespace Migrations
{
struct CreatePostsTable : Migration
{
/*! Filename of the migration file. */
T_MIGRATION
/*! Run the migrations. */
void up() const override
{
Schema::create("posts", [](Blueprint &table)
{
table.id();
table.string(NAME);
table.timestamps();
});
}
/*! Reverse the migrations. */
void down() const override
{
Schema::dropIfExists("posts");
}
};
} // namespace Migrations
Migration classes can be named in two formats, StudlyCase without the datetime prefix and "snake_case" with the datetime prefix. If the StudlyCase name is used then the T_MIGRATION
macro must also be used in the migration class.
Naming with the datetime prefix should look like this.
struct _2014_10_12_000000_create_posts_table : Migration
{
/*! Run the migrations. */
void up() const override
{
//
}
/*! Reverse the migrations. */
void down() const override
{
//
}
};
The StudlyCase naming is preferred. Also the make:migration
command generates migrations in this format.
Setting The Migration Connection
If your migration will be interacting with a database connection other than your application's default database connection, you should set the connection
data member of your migration:
/*! The name of the database connection to use. */
QString connection = QStringLiteral("tinyorm_example");
/*! Run the migrations. */
void up() const override
{
//
}
Running Migrations
To run all of your outstanding migrations, execute the migrate
Tom command:
tom migrate
If you would like to see which migrations have run thus far, you may use the migrate:status
tom command:
tom migrate:status
If you would like to see the SQL statements that will be executed by the migrations without actually running them, you may provide the --pretend
flag to the migrate
command:
tom migrate --pretend
Many tom
commands offer variety of options, you can explore them using the tom list
and tom help
commands. In most cases, these commands and options are self-explanatory.
The tom
command is able to guess the command name and command namespace, eg. tom mig:st
or tom m:rol
, ...
You can pass the -vvv
command-line argument to any command to see all executed SQL queries. 👌
The migrate
Tom command internally calls the migrate:install
command which installs the migration repository table. To uninstall this repository table you can call the migrate:uninstall
.
Forcing Migrations To Run In Production
Some migration operations are destructive, which means they may cause you to lose data. In order to protect you from running these commands against your production database, you will be prompted for confirmation before the commands are executed. To force the commands to run without a prompt, use the --force
flag:
tom migrate --force
Rolling Back Migrations
To roll back the latest migration operation, you may use the rollback
Tom command. This command rolls back the last "batch" of migrations, which may include multiple migration files:
tom migrate:rollback
You may roll back a limited number of migrations by providing the step
option to the rollback
command. For example, the following command will roll back the last five migrations:
tom migrate:rollback --step=5
The migrate:reset
command will roll back all of your application's migrations:
tom migrate:reset
You may roll back a specific "batch" of migrations by providing the batch
option to the rollback
command, where the batch
option corresponds to a batch value within your application's migrations
database table. For example, the following command will roll back all migrations in batch three:
tom migrate:rollback --batch=3
The migrate:reset
command will roll back all of your application's migrations:
tom migrate:reset
The migrate:uninstall
command will uninstall the migration repository table, it optionally accepts the --reset
option to roll back all of your application's migrations:
tom migrate:uninstall --reset
Roll Back & Migrate Using A Single Command
The migrate:refresh
command will roll back all of your migrations and then execute the migrate
command. This command effectively re-creates your entire database:
tom migrate:refresh
You may roll back and re-migrate a limited number of migrations by providing the step
option to the refresh
command. For example, the following command will roll back and re-migrate the last five migrations:
tom migrate:refresh --step=5
Drop All Tables & Migrate
The migrate:fresh
command will drop all tables from the database and then execute the migrate
command:
tom migrate:fresh
The migrate:fresh
command will drop all database tables regardless of their prefix. This command should be used with caution when developing on a database that is shared with other applications.
Tables
Creating Tables
To create a new database table, use the create
method on the Schema
facade. The create
method accepts two arguments: the first is the name of the table, while the second is a lambda expression which receives a Orm::SchemaNs::Blueprint
object that may be used to define the new table:
#include <orm/schema.hpp>
using Orm::Schema;
Schema::create("users", [](Blueprint &table)
{
table.id();
table.string("name");
table.string("email");
table.timestamps();
});
When creating the table, you may use any of the schema builder's column methods to define the table's columns.
Checking For Table / Column Existence
You may check for the existence of a table or column using the hasTable
and hasColumn
methods:
if (Schema::hasTable("users")) {
// The "users" table exists...
}
if (Schema::hasColumn("users", "email")) {
// The "users" table exists and has an "email" column...
}
Database Connection & Table Options
If you want to perform a schema operation on a database connection that is not your application's default connection, use the connection
method or on
alias:
Schema::connection("postgres").create("users", [](Blueprint &table)
{
table.id();
});
In addition, a few other data members and methods may be used to define other aspects of the table's creation. The engine
data member may be used to specify the table's storage engine when using MySQL:
#include <orm/constants.hpp>
using Orm::Constants::InnoDB;
Schema::create("users", [](Blueprint &table)
{
table.engine = InnoDB;
// ...
});
The charset
and collation
data members may be used to specify the character set and collation for the created table when using MySQL:
#include <orm/constants.hpp>
using Orm::Constants::UTF8MB4;
Schema::create("users", [](Blueprint &table)
{
table.charset = UTF8MB4;
table.collation = "utf8mb4_unicode_ci";
// ...
});
The temporary
method may be used to indicate that the table should be "temporary". Temporary tables are only visible to the current connection's database session and are dropped automatically when the connection is closed:
Schema::create("calculations", [](Blueprint &table)
{
table.temporary();
// ...
});
If you would like to add a "comment" to a database table, you may invoke the comment
method on the table instance. Table comments are currently only supported by MySQL and PostgreSQL:
Schema::create("calculations", [](Blueprint &table)
{
table.comment("Business calculations");
// ...
});
Updating Tables
The table
method on the Schema
facade may be used to update existing tables. Like the create
method, the table
method accepts two arguments: the name of the table and a lambda expression that receives a Blueprint
instance you may use to add columns or indexes to the table:
#include <orm/schema.hpp>
Schema::table("users", [](Blueprint &table)
{
table.integer("votes");
});
Renaming / Dropping Tables
To rename an existing database table, use the rename
method:
#include <orm/schema.hpp>
Schema::rename("from", "to");
To drop an existing table, you may use the drop
or dropIfExists
methods:
Schema::drop("users");
Schema::dropIfExists("users");
Renaming Tables With Foreign Keys
Before renaming a table, you should verify that any foreign key constraints on the table have an explicit name in your migration files instead of letting TinyORM assign a convention based name. Otherwise, the foreign key constraint index name will refer to the old table name.
After renaming a table, you can re-create (drop and create again) the foreign key constraints to fix an index name, so it refers to a renamed table.
Columns
Creating Columns
The table
method on the Schema
facade may be used to update existing tables. Like the create
method, the table
method accepts two arguments: the name of the table and a lambda expression that receives a Blueprint
instance you may use to add columns to the table:
#include <orm/schema.hpp>
Schema::table("users", [](Blueprint &table)
{
table.integer("votes");
});
Available Column Types
The schema builder blueprint offers a variety of methods that correspond to the different types of columns you can add to your database tables. Each of the available methods are listed in the table below:
bigIncrements bigInteger binary boolean Char date datetime datetimes datetimeTz decimal Double Enum Float foreignId foreignIdFor foreignUuid geometry geometryCollection id increments integer ipAddress json jsonb lineString longBinary longText macAddress mediumBinary mediumIncrements mediumInteger mediumText multiLineString multiPoint multiPolygon point polygon rememberToken set smallIncrements smallInteger softDeletes softDeletesDatetime softDeletesTz string text time timeTz timestamp timestampTz timestampsTz timestamps tinyBinary tinyIncrements tinyInteger tinyText unsignedBigInteger unsignedDecimal unsignedInteger unsignedMediumInteger unsignedSmallInteger unsignedTinyInteger uuid year
Names of Char
, Double
, Enum
, and Float
column methods are in the CamelCase format to avoid collisions with C++ keywords.
bigIncrements()
The bigIncrements
method creates an auto-incrementing UNSIGNED BIGINT
(primary key) equivalent column:
#include <orm/constants.hpp>
table.bigIncrements(Orm::ID);
bigInteger()
The bigInteger
method creates a BIGINT
equivalent column:
table.bigInteger("votes");
binary()
The binary
method creates a BLOB
equivalent column:
table.binary("photo");
boolean()
The boolean
method creates a BOOLEAN
equivalent column:
table.boolean("confirmed");
Char()
The Char
method creates a CHAR
equivalent column with of a given length:
#include <orm/constants.hpp>
table.Char(Orm::NAME, 100);
date()
The date
method creates a DATE
equivalent column:
table.date("created_at");
datetime()
The datetime
method creates a DATETIME
equivalent column with an optional precision (total digits):
table.datetime("created_at", precision = 0);
datetimes()
The datetimes
method creates created_at
and updated_at
DATETIME
equivalent columns with an optional precision (total digits):
table.datetimes(precision = 0);
datetimeTz()
The datetimeTz
method creates a DATETIME
(with timezone) equivalent column with an optional precision (total digits):
#include <orm/constants.hpp>
table.datetimeTz(Orm::CREATED_AT, precision = 0);
decimal()
The decimal
method creates a DECIMAL
equivalent column with the given precision (total digits) and scale (decimal digits):
table.decimal("amount", precision = 8, scale = 2);
Double()
The Double
method creates a DOUBLE
equivalent column with the given precision (total digits) and scale (decimal digits):
table.Double("amount", 8, 2);
Enum()
The Enum
method creates a ENUM
equivalent column with the given valid values:
table.Enum("difficulty", {"easy", "hard"});
Float()
The Float
method creates a FLOAT
equivalent column with the given precision (total digits) and scale (decimal digits):
table.Float("amount", 8, 2);
foreignId()
The foreignId
method creates an UNSIGNED BIGINT
equivalent column:
table.foreignId("user_id");
foreignIdFor()
The foreignIdFor
method adds a {column}_id UNSIGNED BIGINT
equivalent column for a given model class:
#include "models/user.hpp"
Models::User user;
table.foreignIdFor(User);
foreignUuid()
The foreignUuid
method creates a UUID
equivalent column:
table.foreignUuid("user_id");
geometry()
The geometry
method creates a GEOMETRY
equivalent column:
table.geometry("positions");
geometryCollection()
The geometryCollection
method creates a GEOMETRYCOLLECTION
equivalent column:
table.geometryCollection("positions");
id()
The id
method is an alias of the bigIncrements
method. By default, the method will create an id
column; however, you may pass a column name if you would like to assign a different name to the column:
table.id();
increments()
The increments
method creates an auto-incrementing UNSIGNED INTEGER
equivalent column as a primary key:
table.increments("id");
integer()
The integer
method creates an INTEGER
equivalent column:
table.integer("votes");
ipAddress()
The ipAddress
method creates a VARCHAR(45)
equivalent column:
table.ipAddress("visitor");
json()
The json
method creates a JSON
equivalent column:
table.json("options");
jsonb()
The jsonb
method creates a JSONB
equivalent column:
table.jsonb("options");
lineString()
The lineString
method creates a LINESTRING
equivalent column:
table.lineString("positions");
longBinary()
The longBinary
method creates a LONGBLOB
equivalent column:
table.longBinary("photo");
longText()
The longText
method creates a LONGTEXT
equivalent column:
table.longText("description");
macAddress()
The macAddress
method creates a column that is intended to hold a MAC address. Some database systems, such as PostgreSQL, have a dedicated column type for this type of data. Other database systems will use a string equivalent VARCHAR(17)
column:
table.macAddress("device");
mediumBinary()
The mediumBinary
method creates a MEDIUMBLOB
equivalent column:
table.mediumBinary("photo");
mediumIncrements()
The mediumIncrements
method creates an auto-incrementing UNSIGNED MEDIUMINT
equivalent column as a primary key:
table.mediumIncrements("id");
mediumInteger()
The mediumInteger
method creates a MEDIUMINT
equivalent column:
table.mediumInteger("votes");
mediumText()
The mediumText
method creates a MEDIUMTEXT
equivalent column:
table.mediumText("description");
multiLineString()
The multiLineString
method creates a MULTILINESTRING
equivalent column:
table.multiLineString("positions");
multiPoint()
The multiPoint
method creates a MULTIPOINT
equivalent column:
table.multiPoint("positions");
multiPolygon()
The multiPolygon
method creates a MULTIPOLYGON
equivalent column:
table.multiPolygon("positions");
point()
The point
method creates a POINT
equivalent column:
table.point("position");
polygon()
The polygon
method creates a POLYGON
equivalent column:
table.polygon("position");
rememberToken()
The rememberToken
method creates a nullable, VARCHAR(100)
equivalent column that is intended to store the current "remember me" authentication token:
table.rememberToken();
set()
The set
method creates a SET
equivalent column with the given list of valid values:
table.set("flavors", {"strawberry", "vanilla"});
smallIncrements()
The smallIncrements
method creates an auto-incrementing UNSIGNED SMALLINT
equivalent column as a primary key:
table.smallIncrements("id");
smallInteger()
The smallInteger
method creates a SMALLINT
equivalent column:
table.smallInteger("votes");
softDeletes()
The softDeletes
method adds a nullable deleted_at
TIMESTAMP
equivalent column with an optional precision (total digits). This column is intended to store the deleted_at
timestamp needed for TinyORM's "soft delete" functionality:
table.softDeletes("deleted_at", precision = 0);
softDeletesDatetime()
The softDeletesDatetime
method adds a nullable deleted_at
DATETIME
equivalent column with an optional precision (total digits). This column is intended to store the deleted_at
timestamp needed for TinyORM's "soft delete" functionality:
table.softDeletesDatetime("deleted_at", precision = 0);
softDeletesTz()
The softDeletesTz
method adds a nullable deleted_at
TIMESTAMP
(with timezone) equivalent column with an optional precision (total digits). This column is intended to store the deleted_at
timestamp needed for TinyORM's "soft delete" functionality:
table.softDeletesTz("deleted_at", precision = 0);
string()
The string
method creates a VARCHAR
equivalent column of the given length:
#include <orm/constants.hpp>
table.string(Orm::NAME, 100);
text()
The text
method creates a TEXT
equivalent column:
table.text("description");
time()
The time
method creates a TIME
equivalent column with an optional precision (total digits):
table.time("sunrise", precision = 0);
timeTz()
The timeTz
method creates a TIME
(with timezone) equivalent column with an optional precision (total digits):
table.timeTz("sunrise", precision = 0);
timestamp()
The timestamp
method creates a TIMESTAMP
equivalent column with an optional precision (total digits):
table.timestamp("added_at", precision = 0);
timestampTz()
The timestampTz
method creates a TIMESTAMP
(with timezone) equivalent column with an optional precision (total digits):
table.timestampTz("added_at", precision = 0);
timestampsTz()
The timestampsTz
method creates created_at
and updated_at
TIMESTAMP
(with timezone) equivalent columns with an optional precision (total digits):
table.timestampsTz(precision = 0);
timestamps()
The timestamps
method creates created_at
and updated_at
TIMESTAMP
equivalent columns with an optional precision (total digits):
table.timestamps(precision = 0);
tinyBinary()
The tinyBinary
method creates a TINYBLOB
equivalent column:
table.tinyBinary("photo");
tinyIncrements()
The tinyIncrements
method creates an auto-incrementing UNSIGNED TINYINT
equivalent column as a primary key:
table.tinyIncrements("id");
tinyInteger()
The tinyInteger
method creates a TINYINT
equivalent column:
table.tinyInteger("votes");
tinyText()
The tinyText
method creates a TINYTEXT
equivalent column:
table.tinyText("notes");
unsignedBigInteger()
The unsignedBigInteger
method creates an UNSIGNED BIGINT
equivalent column:
table.unsignedBigInteger("votes");
unsignedDecimal()
The unsignedDecimal
method creates an UNSIGNED DECIMAL
equivalent column with an optional precision (total digits) and scale (decimal digits):
table.unsignedDecimal("amount", precision = 8, scale = 2);
unsignedInteger()
The unsignedInteger
method creates an UNSIGNED INTEGER
equivalent column:
table.unsignedInteger("votes");
unsignedMediumInteger()
The unsignedMediumInteger
method creates an UNSIGNED MEDIUMINT
equivalent column:
table.unsignedMediumInteger("votes");
unsignedSmallInteger()
The unsignedSmallInteger
method creates an UNSIGNED SMALLINT
equivalent column:
table.unsignedSmallInteger("votes");
unsignedTinyInteger()
The unsignedTinyInteger
method creates an UNSIGNED TINYINT
equivalent column:
table.unsignedTinyInteger("votes");
uuid()
The uuid
method creates a UUID
equivalent column:
table.uuid("id");
year()
The year
method creates a YEAR
equivalent column:
table.year("birth_year");
Column Modifiers
In addition to the column types listed above, there are several column "modifiers" you may use when adding a column to a database table. For example, to make the column "nullable", you may use the nullable
method:
#include <orm/schema.hpp>
Schema::table("users", [](Blueprint &table)
{
table.string("email").nullable();
});
The following table contains all of the available column modifiers. This list does not include index modifiers:
Modifier | Description |
---|---|
.after("column") | Place the column "after" another column (MySQL). |
.autoIncrement() | Set INTEGER columns as auto-incrementing (primary key). |
.charset("utf8mb4") | Specify a character set for the column (MySQL). |
.collation("utf8mb4_unicode_ci") | Specify a collation for the column (MySQL/PostgreSQL/SQL Server). |
.comment("my comment") | Add a comment to a column (MySQL / PostgreSQL). Special characters are escaped. |
.defaultValue(value) | Specify a "default" value for the column. Special characters are escaped. |
.first() | Place the column "first" in the table (MySQL). |
.from(integer) | Set the starting value of an auto-incrementing field, an alias for startingValue() (MySQL / PostgreSQL). |
.invisible() | Make the column "invisible" to SELECT * queries (MySQL). |
.nullable(value = true) | Allow NULL values to be inserted into the column. |
.startingValue(integer) | Set the starting value of an auto-incrementing field (MySQL / PostgreSQL). |
.storedAs(expression) | Create a stored generated column (MySQL / PostgreSQL). |
.unsigned() | Set INTEGER columns as UNSIGNED (MySQL). |
.useCurrent() | Set TIMESTAMP columns to use CURRENT_TIMESTAMP as default value. |
.useCurrentOnUpdate() | Set TIMESTAMP columns to use CURRENT_TIMESTAMP when a record is updated. |
.virtualAs(expression) | Create a virtual generated column (MySQL). |
.generatedAs(expression) | Create an identity column with specified sequence options (PostgreSQL). |
.always() | Defines the precedence of sequence values over input for an identity column (PostgreSQL). |
.isGeometry() | Set spatial column type to geometry - the default type is geography (PostgreSQL). |
Default Expressions
The defaultValue
modifier accepts a value or an Orm::Query::Expression
instance. Using an Expression
instance will prevent TinyORM from wrapping the value in quotes and allow you to use database-specific functions. One situation where this is particularly useful is when you need to assign default values to JSON columns:
#include <orm/schema.hpp>
using Orm::Query::Expression;
Schema::create("flights", [](Blueprint &table)
{
table.id();
table.json("detail").defaultValue(Expression("(JSON_ARRAY('none'))"));
table.timestamps();
});
Support for default expressions depends on your database driver, database version, and the field type. Please refer to your database's documentation.
You can obtain an Orm::Query::Expression
using the DB::raw
method if you have access to the DB
facade.
Column Order
When using the MySQL database, the after
method may be used to add columns after an existing column in the schema:
table.after("password", [](Blueprint &table)
{
table.string("address_line1");
table.string("address_line2");
table.string("city");
});
Modifying Columns
The change
method allows you to modify the type and attributes of existing columns. For example, you may wish to increase the size of a string
column. To see the change
method in action, let's increase the size of the name
column from 25 to 50. To accomplish this, we simply define the new state of the column and then call the change
method:
#include <orm/schema.hpp>
Schema::table("users", [](Blueprint &table)
{
table.string("name", 50).change();
});
When modifying a column, you must explicitly include all of the modifiers you want to keep on the column definition - any missing attribute will be dropped. For example, to retain the unsigned
, default
, and comment
attributes, you must call each modifier explicitly when changing the column:
Schema::table("users", [](Blueprint &table)
{
table.integer("votes").isUnsigned().defaultValue(1).comment("my comment").change();
});
The change
method and modifying columns is not implemented for the SQLite
database because it doesn't support modifying columns out of the box.
Renaming Columns
To rename a column, you may use the renameColumn
method provided by the schema builder blueprint:
Schema::table("users", [](Blueprint &table)
{
table.renameColumn("from", "to");
});
Renaming Columns On Legacy Databases
Renaming columns is not supported if you are running a database installation older than one of the following releases:
- MySQL
<8.0.3
- MariaDB
<10.5.2
- SQLite
<3.25.0
Dropping Columns
To drop a column, you may use the dropColumn
method on the schema builder blueprint:
Schema::table("users", [](Blueprint &table)
{
table.dropColumn("votes");
});
You may drop multiple columns from a table by passing a QVector<QString>
of column names to the dropColumns
method, the dropColumns
method also provides parameter pack overload:
Schema::table("users", [](Blueprint &table)
{
table.dropColumns({"votes", "avatar", "location"});
// Parameter pack overload
table.dropColumns("votes", "avatar", "location");
});
The SQLite prior to v3.35.0
doesn't support dropping columns using the ALTER TABLE DROP COLUMN
, dropping columns was added in the SQLite v3.35.0
as is described in the release notes.
Available Command Aliases
TinyORM provides several convenient methods related to dropping common types of columns. Each of these methods is described in the table below:
Command | Description |
---|---|
table.dropRememberToken(); | Drop the remember_token column. |
table.dropSoftDeletes(); | Drop the deleted_at column. |
table.dropSoftDeletesDatetime(); | Alias of dropSoftDeletes() method. |
table.dropSoftDeletesTz(); | Alias of dropSoftDeletes() method. |
table.dropTimestamps(); | Drop the created_at and updated_at columns. |
table.dropTimestampsTz(); | Alias of dropTimestamps() method. |
table.dropDatetimes(); | Alias of dropTimestamps() method. |
Indexes
Creating Indexes
The TinyORM schema builder supports several types of indexes. The following example creates a new email
column and specifies that its values should be unique. To create the index, we can chain the unique
method onto the column definition:
#include <orm/schema.hpp>
Schema::table("users", [](Blueprint &table)
{
table.string("email").unique();
});
Alternatively, you may create the index after defining the column. To do so, you should call the unique
method on the schema builder blueprint. This method accepts the name of the column that should receive a unique index:
table.unique("email");
You may even pass a QVector<QString>
of columns to an index method to create a compound (or composite) index:
table.index({"account_id", "created_at"});
When creating an index, TinyORM will automatically generate an index name based on the table, column names, and the index type (eg. users_email_unique), but you may pass a second argument to the method to specify the index name yourself:
table.unique("email", "unique_email");
Available Index Types
TinyORM's schema builder blueprint class provides methods for creating each type of index supported by TinyORM. Each index method accepts an optional second argument to specify the name of the index. If omitted, the name will be derived from the names of the table and column(s) used for the index, as well as the index type (eg. users_email_fulltext). Each of the available index methods is described in the table below:
Command | Description |
---|---|
table.primary("id"); | Adds a primary key. |
table.primary({"id", "parent_id"}); | Adds composite keys. |
table.unique("email"); | Adds a unique index. |
table.index("state"); | Adds an index. |
table.fullText("body"); | Adds a full text index (MySQL/PostgreSQL). |
table.fullText("body").language("english"); | Adds a full text index of the specified language (PostgreSQL). |
table.spatialIndex("location"); | Adds a spatial index (except SQLite). |
Index Lengths & MySQL / MariaDB
By default, TinyORM uses the utf8mb4
character set. If you are running a version of MySQL older than the 5.7.7 release or MariaDB older than the 10.2.2 release, you may need to manually configure the default string length generated by migrations in order for MySQL to create indexes for them. You may configure the default string length by calling the Schema::defaultStringLength
method:
#include <orm/schema.hpp>
Schema::defaultStringLength(191);
Alternatively, you may enable the innodb_large_prefix
option for your database (enabled by default in >=MySQL 5.7.7). Refer to your database's documentation for instructions on how to properly enable this option.
Renaming Indexes
To rename an index, you may use the renameIndex
method provided by the schema builder blueprint. This method accepts the current index name as its first argument and the desired name as its second argument:
table.renameIndex("from", "to");
Dropping Indexes
To drop an index, you must specify the index's name. By default, TinyORM automatically assigns an index name based on the table name, the name of the indexed column, and the index type (eg. users_email_unique). Here are some examples:
Command | Description |
---|---|
table.dropPrimary("users_id_primary"); | Drop a primary key from the "users" table. |
table.dropUnique("users_email_unique"); | Drop a unique index from the "users" table. |
table.dropIndex("geo_state_index"); | Drop a basic index from the "geo" table. |
table.dropFullText("posts_body_fulltext"); | Drop a full text index from the "posts" table. |
.dropSpatialIndex("geo_location_spatialindex"); | Drop a spatial index from the "geo" table (except SQLite). |
I may also drop indexes by a column name or column names for composite keys, if you pass a QVector<QString>
of columns into a method that drops indexes, the conventional index name will be generated based on the table name, columns, and index type:
Schema::table("geo", [](Blueprint &table)
{
table.dropIndex({"state"}); // Drops index 'geo_state_index'
});
Foreign Key Constraints
TinyORM also provides support for creating foreign key constraints, which are used to force referential integrity at the database level. For example, let's define a user_id
column on the posts
table that references the id
column on a users
table:
#include <orm/schema.hpp>
using Orm::Constants::ID;
Schema::table("posts", [](Blueprint &table)
{
table.unsignedBigInteger("user_id");
table.foreign("user_id").references(ID).on("users");
});
Since this syntax is rather verbose, TinyORM provides additional, terser methods that use conventions to provide a better developer experience. When using the foreignId
method to create your column, the example above can be rewritten like so:
Schema::table("posts", [](Blueprint &table)
{
table.foreignId("user_id").constrained();
});
The foreignId
method creates an UNSIGNED BIGINT
equivalent column, while the constrained
method will use conventions to determine the table and column name being referenced. If your table name does not match TinyORM's conventions, you may specify the table name by passing it as an argument to the constrained
method:
Schema::table("posts", [](Blueprint &table)
{
table.foreignId("user_id").constrained("users");
});
You may also specify the desired action for the "on delete" and "on update" properties of the constraint:
#include <orm/constants.hpp>
using Orm::SchemaNs::Constants::Cascade;
table.foreignId("user_id")
.constrained()
.onUpdate("cascade")
.onDelete(Cascade);
An alternative, expressive syntax is also provided for these actions:
Method | Description |
---|---|
table.cascadeOnUpdate(); | Updates should cascade. |
table.restrictOnUpdate(); | Updates should be restricted. |
table.cascadeOnDelete(); | Deletes should cascade. |
table.restrictOnDelete(); | Deletes should be restricted. |
table.nullOnDelete(); | Deletes should set the foreign key value to null. |
Any additional column modifiers must be called before the constrained
method:
table.foreignId("user_id")
.nullable()
.constrained();
Dropping Foreign Keys
To drop a foreign key, you may use the dropForeign
method, passing the name of the foreign key constraint to be deleted as an argument. Foreign key constraints use the same naming convention as indexes. In other words, the foreign key constraint name is based on the name of the table and the columns in the constraint, followed by a "_foreign" suffix:
table.dropForeign("posts_user_id_foreign");
Alternatively, you may pass a QVector<QString>
containing the column name that holds the foreign key to the dropForeign
method. The QVector
will be converted to a foreign key constraint name using TinyORM's constraint naming conventions:
table.dropForeign({"user_id"});
Toggling Foreign Key Constraints
You may enable or disable foreign key constraints within your migrations by using the following methods:
Schema::enableForeignKeyConstraints();
Schema::disableForeignKeyConstraints();
Schema::withoutForeignKeyConstraints([]
{
// Constraints disabled within this lambda expression...
});
The SQLite disables foreign key constraints by default. When using SQLite, make sure to enable foreign key support in your database configuration before attempting to create them in your migrations. In addition, SQLite only supports creating foreign keys when creating tables and not when tables are altered.